1.實驗目的
通過編寫多進程程序,使讀者熟練掌握fork()、exec()、wait()和waitpid()等函數的使用,進一步理解在Linux中多進程編程的步驟。
2.實驗內容
該實驗有3個進程,其中一個為父進程,其余兩個是該父進程創建的子進程,其中一個子進程運行“ls -l”指令,另一個子進程在暫停5s后異常退出。父進程先用阻塞方式等待第一個子進程的結束,然后用非阻塞方式等待另一個子進程的退出,待收集到第二個子進程結束的信息后,父進程就返回。
3.實驗步驟
(1)畫出該實驗流程圖。該實驗流程圖如圖1所示。
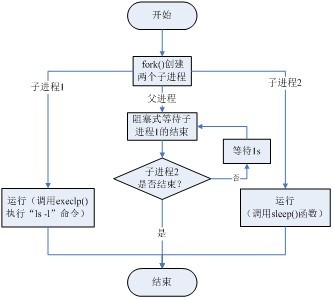 圖1 實驗流程圖
(2)實驗源代碼。先看一下下面的代碼,這個程序能得到我們所希望的結果嗎?它的運行會產生幾個進程?請讀者回憶一下fork()調用的具體過程。
/* multi_proc_wrong.c */
#include <stdio.h>
#include <stdlib.h>
#include <sys/types.h>
#include <unistd.h>
#include <sys/wait.h>
int main(void)
{
pid_t child1, child2, child;
/* 創建兩個子進程 */
child1 = fork();
child2 = fork();
/* 子進程1的出錯處理 */
if (child1 == -1)
{
printf("Child1 fork error\n");
exit(1);
}
/* 在子進程1中調用execlp()函數 */
else if (child1 == 0)
{
printf("In child1: execute 'ls -l'\n");
if (execlp("ls", "ls", "-l", NULL) < 0)
{
printf("Child1 execlp error\n");
}
}
/* 子進程2的出錯處理 */
if (child2 == -1)
{
printf("Child2 fork error\n");
exit(1);
}
/* 在子進程2中使其暫停5s */
else if( child2 == 0 )
{
printf("In child2: sleep for 5 seconds and then exit\n");
sleep(5);
exit(0);
}
/* 在父進程中等待兩個子進程的退出 */
else
{
printf("In father process:\n");
child = waitpid(child1, NULL, 0); /* 阻塞式等待 */
if (child == child1)
{
printf("Get child1 exit code\n");
}
else
{
printf("Error occured!\n");
}
do
{
child = waitpid(child2, NULL, WNOHANG);/* 非阻塞式等待 */
if (child == 0)
{
printf("The child2 process has not exited!\n");
sleep(1);
}
} while (child == 0);
if (child == child2)
{
printf("Get child2 exit code\n");
}
else
{
printf("Error occured!\n");
}
}
exit(0);
}
編譯和運行以上代碼,并觀察其運行結果。它的結果是我們所希望得到的嗎?
看完前面的代碼后,再觀察下面的代碼,比較一下它們之間有什么區別,看看會解決哪些問題。
/* multi_proc.c */
#include <stdio.h>
#include <stdlib.h>
#include <sys/types.h>
#include <unistd.h>
#include <sys/wait.h>
int main(void)
{
pid_t child1, child2, child;
/* 創建兩個子進程 */
child1 = fork();
/* 子進程1的出錯處理 */
if (child1 == -1)
{
printf("Child1 fork error\n");
exit(1);
}
/* 在子進程1中調用execlp()函數 */
else if (child1 == 0)
{
printf("In child1: execute 'ls -l'\n");
if (execlp("ls", "ls", "-l", NULL) < 0)
{
printf("Child1 execlp error\n");
}
}
/* 在父進程中再創建進程2,然后等待兩個子進程的退出 */
else
{
child2 = fork();
/* 子進程2的出錯處理 */
if (child2 == -1)
{
printf("Child2 fork error\n");
exit(1);
}
/* 在子進程2中使其暫停5s */
else if(child2 == 0)
{
printf("In child2: sleep for 5 seconds and then exit\n");
sleep(5);
exit(0);
}
printf("In father process:\n");
…(以下部分與前面程序的父進程執行部分相同)
}
exit(0);
}
(3)首先在宿主機上編譯、調試該程序:
$ gcc multi_proc.c –o multi_proc(或者使用Makefile)
(4)在確保沒有編譯錯誤后,使用交叉編譯該程序:
$ arm-linux-gcc multi_proc.c –o multi_proc (或者使用Makefile)
(5)將生成的可執行程序下載到目標板上運行。
4.實驗結果
在目標板上運行的結果如下(具體內容與各自的系統有關):
$ ./multi_proc
In child1: execute 'ls -l' /* 子進程1的顯示, 以下是“ls –l”的運行結果 */
total 28
-rwxr-xr-x 1 david root 232 2008-07-18 04:18 Makefile
-rwxr-xr-x 1 david root 8768 2008-07-20 19:51 multi_proc
-rw-r--r-- 1 david root 1479 2008-07-20 19:51 multi_proc.c
-rw-r--r-- 1 david root 3428 2008-07-20 19:51 multi_proc.o
-rw-r--r-- 1 david root 1463 2008-07-20 18:55 multi_proc_wrong.c
In child2: sleep for 5 seconds and then exit /* 子進程2的顯示 */
In father process: /* 以下是父進程顯示 */
Get child1 exit code /* 表示子進程1結束(阻塞等待) */
The child2 process has not exited! /* 等待子進程2結束(非阻塞等待) */
The child2 process has not exited!
The child2 process has not exited!
The child2 process has not exited!
The child2 process has not exited!
Get child2 exit code /* 表示子進程2終于結束了*/
本文選自華清遠見嵌入式培訓教材《從實踐中學嵌入式Linux應用程序開發》
熱點鏈接:
1、Linux下多進程編程之exec函數語法及使用實例
2、Linux下多進程編程之fork()函數語法
3、Linux下多進程編程之fork()函數說明
4、Linux守護進程
5、wait()和waitpid()函數
更多新聞>> |